Translating Text in Real-Time with JavaScript Using Free Google Translate API
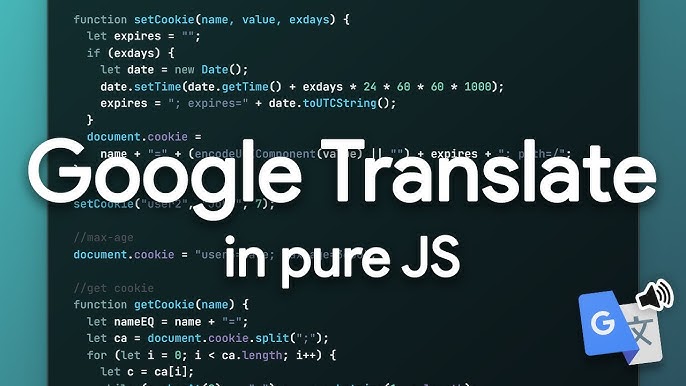
As the world becomes more interconnected, the need for real-time translation in web applications is skyrocketing. Whether you’re building a chat app, an e-commerce site, or a global news platform, providing real-time translation can make your app accessible to a much wider audience. In this blog, I’ll walk you through how to implement a quick and easy text translation feature using JavaScript and Google’s free Translate API.
Let’s take a look at the core concept and the actual code to translate text on the fly, seamlessly integrating it into your real-time applications.
Why Use Google Translate API?
Google Translate has become the go-to tool for translating text across different languages, supporting over 100 languages worldwide. It’s fast, reliable, and—best of all—it’s free to use (within reasonable limits). This makes it an excellent choice for developers who want to integrate real-time translation into their applications without having to worry about extensive setup or costs.
Now, let’s break down how you can implement this in JavaScript.
Getting Started: The Basic Structure
The basic idea behind using Google’s free Translate API is simple. You send a request to Google’s servers, passing along the text you want translated, along with the source and target languages. Google then sends back the translated text, which you can display directly in your application.
Below is the JavaScript code that does exactly that:
// Function to perform the translation
async function translateText() {
try {
// Define the language codes and text to be translated
const inputLanguage = 'en'; // Source language code (e.g., 'en' for English)
const outputLanguage = 'fr'; // Target language code (e.g., 'fr' for French)
const inputText = 'Hello, how are you?'; // Text to be translated
// Construct the URL for the Google Translate API request
const url = `https://translate.googleapis.com/translate_a/single?client=gtx&sl=${inputLanguage}&tl=${outputLanguage}&dt=t&q=${encodeURIComponent(inputText)}`;
const response = await fetch(url);
if (!response.ok) {
throw new Error('Network response was not ok');
}
const json = await response.json();
// Extract the translated text from the JSON response
const translatedText = json[0].map((item) => item[0]).join("");
console.log('Translated Text:', translatedText);
} catch (error) {
console.error('Translation error:', error);
}
}
// Run the translation function
translateText();
Explaining the Code in Detail
Let’s break the code down step by step so you can understand how it works and why it’s structured this way.
Setting Up the Languages and Text to Translate
At the beginning of the translateText function, we define three key variables:
const inputLanguage = 'en'; // Source language code
const outputLanguage = 'fr'; // Target language code
const inputText = 'Hello, how are you?'; // Text to be translated
Building the API Request URL
Next, we construct the URL that will be used to send the request to Google’s translation servers:
const url = `https://translate.googleapis.com/translate_a/single?client=gtx&sl=${inputLanguage}&tl=${outputLanguage}&dt=t&q=${encodeURIComponent(inputText)}`;
Here’s what’s happening in the URL:
By dynamically inserting the language codes and text, this URL can be adapted for translating virtually any string of text into any language
Sending the API Request
The fetch() method is used to send an asynchronous HTTP request to the Google Translate API:
const response = await fetch(url);
This sends the translation request to Google’s servers and waits for a response. If the response is successful (i.e., no network errors), the function proceeds to process the returned data.
Handling the API Response
Once the response is received, we need to extract the translated text from the JSON format returned by Google:
const json = await response.json();
const translatedText = json[0].map((item) => item[0]).join("");
The response comes back as a deeply nested array, so we use map() to iterate through the array and extract the translated text from the appropriate location. We then join all the text fragments together into a single string.
Error Handling
Any time you’re working with API requests, you should always include error handling. If the network request fails for some reason (e.g., a bad internet connection or an issue with the API), this block will catch the error and log it to the console:
catch (error) {
console.error('Translation error:', error);
}
This ensures that your application won’t crash unexpectedly, and you can gracefully handle any errors that may occur.
Real-Time Translation in Web Applications
Now that you have the basic code, imagine how powerful this can be when integrated into a real-time application. For example, you could:
Since Google’s API responds quickly, you can easily handle translation in real-time without significant delays. However, keep in mind that the free version of the Google Translate API has some usage limitations. If your app scales up and starts hitting those limits, you may want to explore using Google’s paid Cloud Translation API.
This solution is perfect for lightweight translation needs, offering a quick and straightforward way to bridge language barriers in your app. Whether you’re working on a small project or building the next big global platform, this setup can help you provide a better user experience for non-English speaking users.
Feel free to take this code and modify it to suit your needs—whether that means changing the input languages, translating user-generated content, or building a real-time multi-lingual chat platform. The possibilities are endless!
Happy coding!